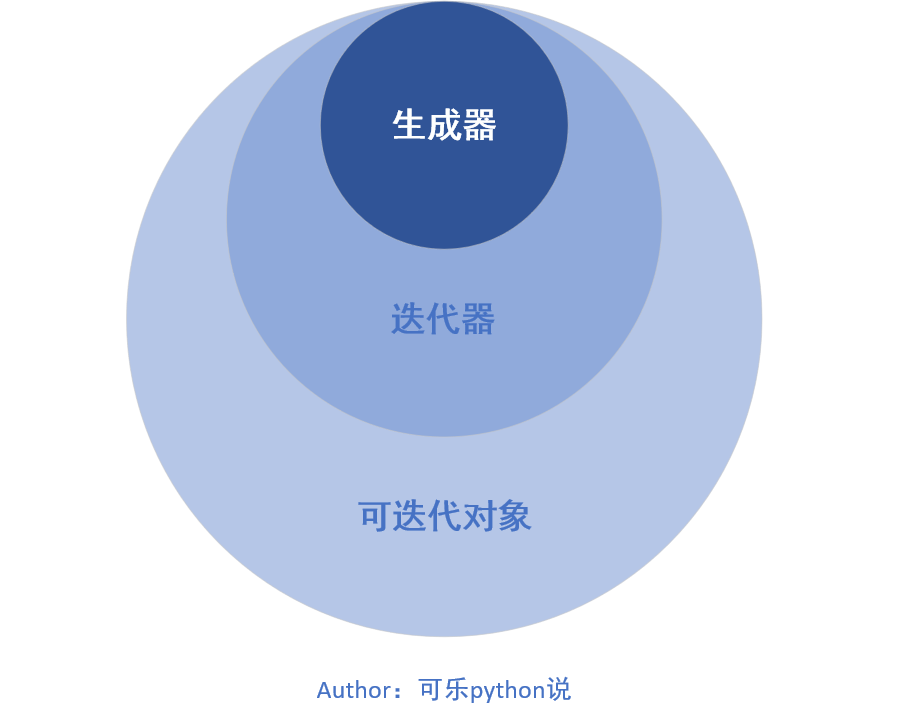
可迭代对象
1 2 3 4
| 实现了__iter__的对象就是可迭代对象 class IsIterable: def __iter__(self): return self
|
迭代器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| 实现了 __ iter __ +__ next __ >>> class MyIterator: """ 迭代器类 Author:可乐python说 """ def __init__(self): self.num = 0 def __iter__(self): return self def __next__(self): return_num = self.num # 只要值大于等于6,就停止迭代 if return_num >= 6: raise StopIteration self.num += 2 return return_num
>>> my_iterator = MyIterator() >>> next(my_iterator) 0 >>> next(my_iterator) 2 >>> next(my_iterator) 4 >>> next(my_iterator) Traceback (most recent call last): File "<input>", line 1, in <module> StopIteration
|
生成器
1 2
| 特殊的迭代器 不需要实现 __ iter __ +__ next __ 又可以实现相同的作用 ,有以下两种方式
|
生成器函数
借助yield实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| >>> def my_generator(): my_num = 0 while my_num < 5: yield my_num my_num += 1
>>>generator_ = my_generator() >>> next(generator_) 0 >>> next(generator_) 1 >>> next(generator_) 2 >>> next(generator_) 3 >>> next(generator_) 4 >>> next(generator_) # 终止迭代则会抛出 StopIteration 异常 Traceback (most recent call last): File "<input>", line 1, in <module> StopIteration
|
生成器表达式
使用 ()
包裹,而不是【】
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| g = ((i)**2 for i in range(5)) print (next(g)) print (next(g)) print (next(g)) print (next(g)) print (next(g)) print (next(g)) print (next(g))
0 1 4 9 16 Traceback (most recent call last): File "d:\python\test.py", line 7, in <module> print (next(g)) StopIteration
|
优势
1 2 3 4 5 6 7 8 9 10 11
| import sys
my_list = [i for i in range(1000000)] print( "列表消耗的内存:{}".format(sys.getsizeof(my_list)))
my_generator = (i for i in range(1000000)) print("生成器消耗的内存:{}".format(sys.getsizeof(my_generator)))
列表消耗的内存:8448728 生成器消耗的内存:112
|
参考
https://www.runoob.com/python3/python3-iterator-generator.html
https://kelepython.readthedocs.io/zh/latest/c01/c01_11.html